TypeScript ASCII Art!
Monday 8 January 2024
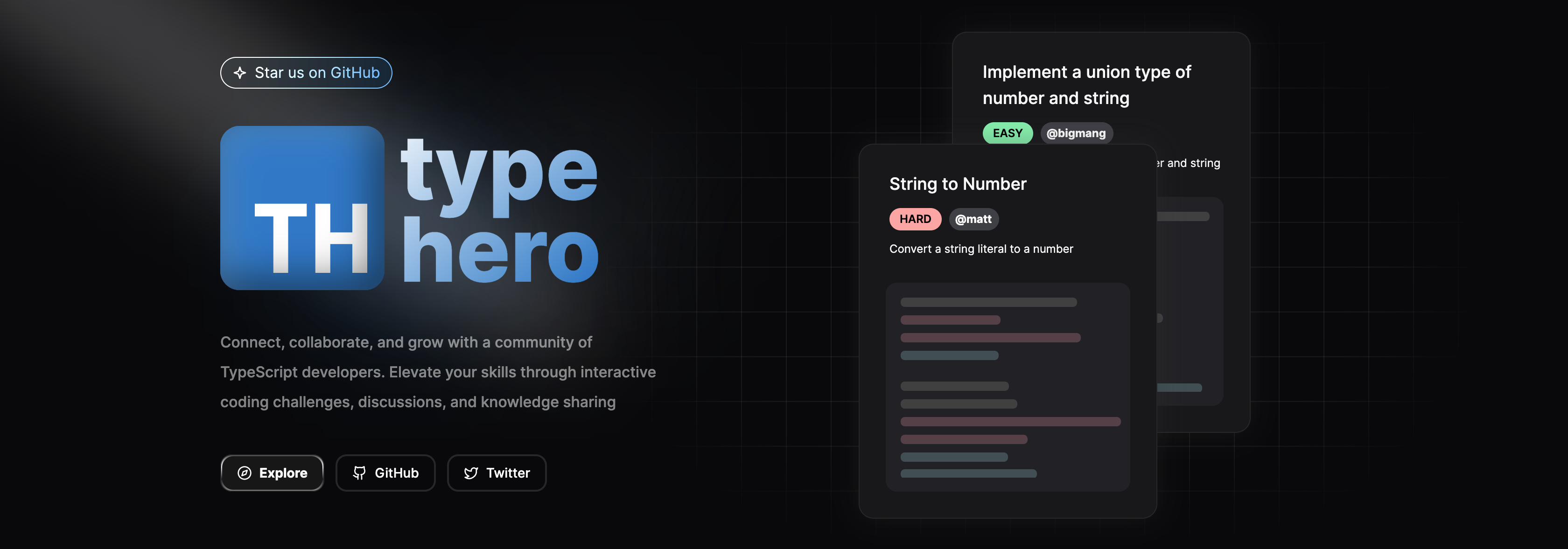
Typehero - advent of typescript solution for day 20!
Day 20 of the typehero advent of code involved converting regular strings to ascii art.
The Problem
Meaning you would need to convert test_0_actual to test_0_expected
the letters are already provided to you in a record with the type signature of Record<string, [string, string, string]>
which has
- key - string to be converted
- value - top, middle and bottom of the ascii version of the key
The Idea
This is a complicated type that requires a few utility types to work
- Generate - accepts a string and uses it to create a tuple containing the corresponding
Letters
tuples - GetIndex - accepts a a tuple of
Letters
tuples and gets a specific index in the tuple - Join - accepts a tuple of
Letters
tuples and adds them to each other based on their index - ToAsciiArt - the final type which wraps up all the other types and is used by the typehero challenge